How to Fix Hydration Mismatch Error in Next js?
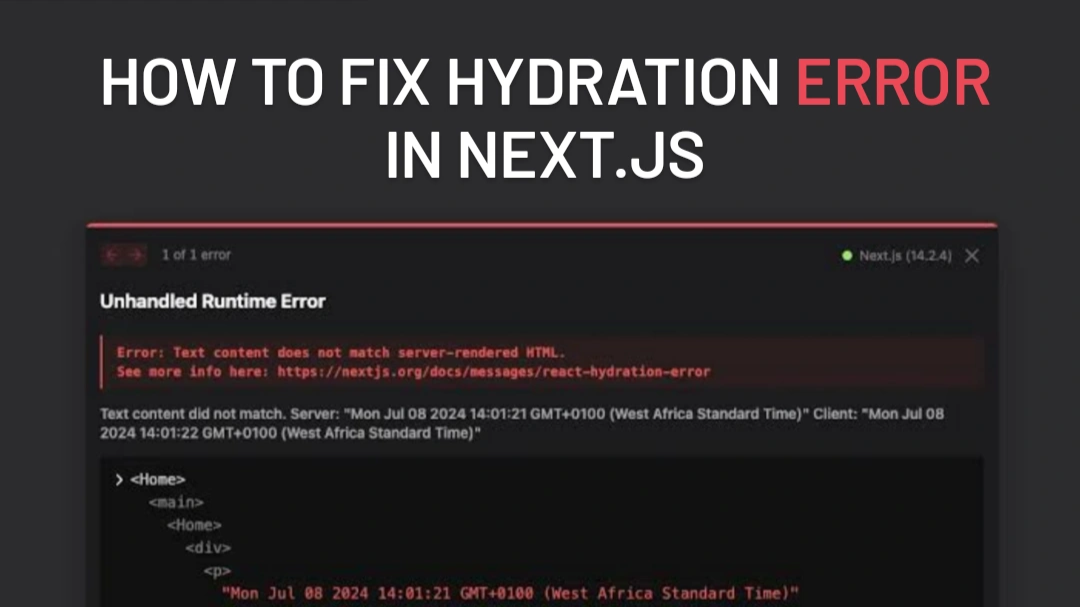
Hydration errors are among the most frustrating issues developers face when working with Next.js.
One moment your app runs fine during development, and the next, you see warnings like:
These hydration mismatches can break the seamless user experience, hurt SEO, and make debugging a nightmare.
In this guide, we'll explore what hydration errors are, why they occur, common scenarios, and practical ways to fix them.
What is a Hydration Error?
In Next.js (and React in general), hydration is the process where React takes over the static HTML generated by the server and attaches event listeners and dynamic functionality on the client side.
This ensures your app is interactive immediately after the page loads.
A hydration error occurs when there's a mismatch between what was rendered on the server (Server-Side Rendering or SSR) and what React expects on the client during hydration.
The DOM must be identical on both ends. Any discrepancy leads to hydration warnings or errors.
Common Causes of Hydration Mismatches
1. Non-Deterministic Rendering (Random Values)
If your component uses Math.random()
or Date.now()
during SSR, the output will differ between server and client.
2. Client-Specific APIs or Window Access
Accessing window
, localStorage
, or other browser-only objects on the server will break hydration.
3. Time-Sensitive Content
Displaying current time, countdowns, or live data without synchronization.
Fixes & Workarounds
1. Conditional Rendering with
useEffect
useEffect
only runs on the client after hydration. This makes it a safe space to use browser-only APIs.
2. Dynamic Imports with ssr: false
Next.js lets you load components only on the client using dynamic
imports.
3. Client-Only Components
Wrap sensitive logic in a "ClientOnly" component that renders children after hydration.
Usage:
4. Guarding Code with typeof window
You can also prevent SSR issues by wrapping code that accesses browser APIs.
Best Practices to Avoid Hydration Issues
1. Avoid Side Effects in Render
Never run side effects (like logging, timers, or fetching data) directly inside your render method.
2. Consistent Server & Client Output
Ensure that any code rendered both on the server and client produces the same output.
3. Use useEffect
Wisely
Always move browser-dependent logic into useEffect
or useLayoutEffect
.
4. Be Mindful with Third-Party Libraries
Some UI libraries manipulate the DOM in unexpected ways. Wrap them in dynamic()
with ssr: false
if needed.
5. Keep Server Logic Pure
Make sure the server-side rendered components don’t depend on variables that change per client unless fetched explicitly.
Conclusion
Hydration errors in Next.js can be intimidating at first, but they’re easily manageable with the right patterns. Always remember: what the server sees,
The client should see too—until it hydrates. Whether you use useEffect
, dynamic imports, or client-only components, the key is to isolate browser-only logic from the SSR process.
By following these guidelines, you'll not only fix hydration issues but also build more robust, predictable, and performant Next.js applications.
If this guide helped you, checkout my other blogs https://www.jigsdev.xyz/blogs/nextjs-performance-optimization