How to Integrate Razorpay in Next js 15 for UPI Payments
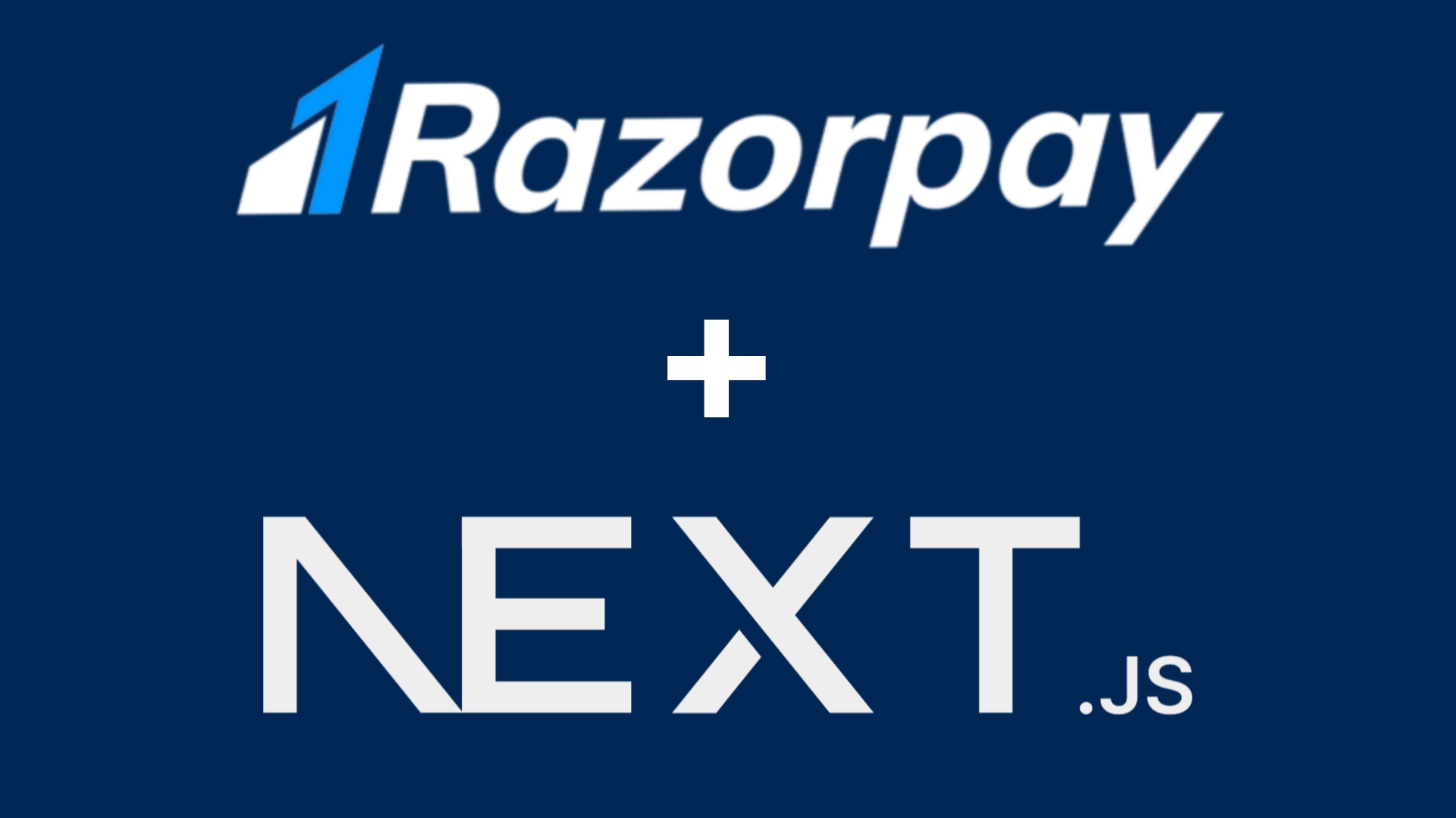
Integrating a payment gateway like Razorpay into your Next.js 15 project is essential if you're building a real-world application that requires accepting money from users—whether it’s for subscriptions, e-commerce, donations, or services.
Razorpay supports various payment methods, including UPI, making it a go-to choice for developers in India.
In this tutorial, you'll learn how to integrate Razorpay's UPI payments into a Next.js 15 app using the App Router.
Why Use Razorpay with Next.js 15?
- Razorpay offers quick UPI payments—a must-have for Indian customers.
- You can provide a seamless in-app payment experience.
- It supports secure transactions and real-time payment confirmations.
- With Next.js 15’s App Router and server actions, integrating APIs is even easier.
Prerequisites
Make sure you have the following ready:
- A Next.js 15 project set up (
npx create-next-app@latest
) - Razorpay account and access to API keys
- Node.js and npm/yarn installed
- Basic knowledge of React and TypeScript (optional)
Step 1: Set Up Razorpay Keys
- Go to the Razorpay Dashboard.
- Navigate to Settings → API Keys and generate your key pair.
- In your Next.js project, create a
.env.local
file and add:
Don't expose your secret key on the frontend.
Step 2: Install Razorpay SDK
Install Razorpay’s Node.js SDK for order creation:
Step 3: Create Razorpay Order API (App Router)
In Next.js 15 App Router, APIs live in the app/api/
folder. Create this file:
app/api/razorpay/route.ts
Step 4: Include Razorpay Script
In your layout file, add the Razorpay script tag:
app/layout.tsx
Step 5: Create a Payment Button Component
components/PaymentButton.tsx
Step 6: Use It in a Page
In any page (e.g., app/page.tsx
), use the payment button:
Summary and Key Takeaways
You’ve now learned how to integrate Razorpay UPI payments in a modern Next.js 15 application using the App Router.
What you did:
- Generated Razorpay API keys and stored them securely.
- Created a backend route for order generation.
- Added Razorpay Checkout script.
- Built a reusable UPI payment button on the frontend.
This setup is flexible and can be extended to include features like success pages, webhooks, and payment verification.