Back to blogs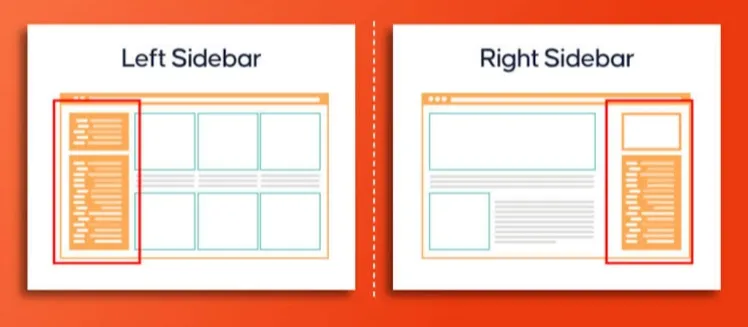
How to Create a Sticky Left and Right Sidebar with a Scrollable Center Section (HTML, CSS, and Tailwind JSX Example)
March 10, 2025
5 min read
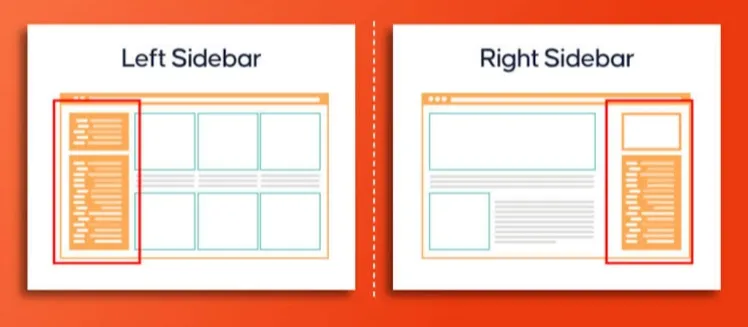
Introduction
In this blog, we’ll explore how to create a layout with sticky left and right sidebars and a scrollable center section. This layout is useful for dashboards, blogs, and other web applications where you want to keep the sidebars fixed while the center content scrolls independently.
Key Points:
- Sticky Sidebar: The left and right sidebars will remain fixed even when scrolling.
- Scrollable Center Section: The center section will be scrollable, allowing users to focus on the content without moving the sidebars.
- Responsive Design: This layout will be fully responsive with Tailwind CSS classes.
HTML & CSS Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sticky Sidebar Layout</title>
<style>
body, html {
margin: 0;
padding: 0;
height: 100%;
}
.container {
display: flex;
height: 100%;
}
.sidebar-left,
.sidebar-right {
position: sticky;
top: 0;
height: 100vh;
width: 200px;
background: #333;
color: white;
padding: 20px;
box-sizing: border-box;
}
.center-content {
flex-grow: 1;
overflow-y: auto;
padding: 20px;
background: #f4f4f4;
height: 100vh;
}
</style>
</head>
<body>
<div class="container">
<div class="sidebar-left">Left Sidebar</div>
<div class="center-content">
<h1>Main Content</h1>
<p>Scroll down to see the sticky effect...</p>
</div>
<div class="sidebar-right">Right Sidebar</div>
</div>
</body>
</html>
JSX with Tailwind CSS Example
import React from "react";
const StickyLayout = () => {
return (
<div className="flex h-screen">
<div className="sticky top-0 w-64 bg-gray-800 text-white p-4">Left Sidebar</div>
<div className="flex-grow overflow-y-auto bg-gray-100 p-4">
<h1>Main Content</h1>
<p>Scroll down to see the sticky effect...</p>
</div>
<div className="sticky top-0 w-64 bg-gray-800 text-white p-4">Right Sidebar</div>
</div>
);
};
export default StickyLayout;
Explanation:
- Flexbox: We use
flex
to create a layout with the sidebars and center content. - Sticky Positioning:
sticky top-0
keeps the sidebars fixed at the top of the viewport as you scroll. - Overflowing Content:
overflow-y-auto
on the center content allows it to scroll independently of the sidebars.
This layout is both functional and responsive, and Tailwind's utility-first classes make it quick and easy to implement.
HTMLCSSTailwindReactWeb Development