How to Optimize Performance of a Next.js App: Best Practices for Speed and SEO (Next.js 15.2)
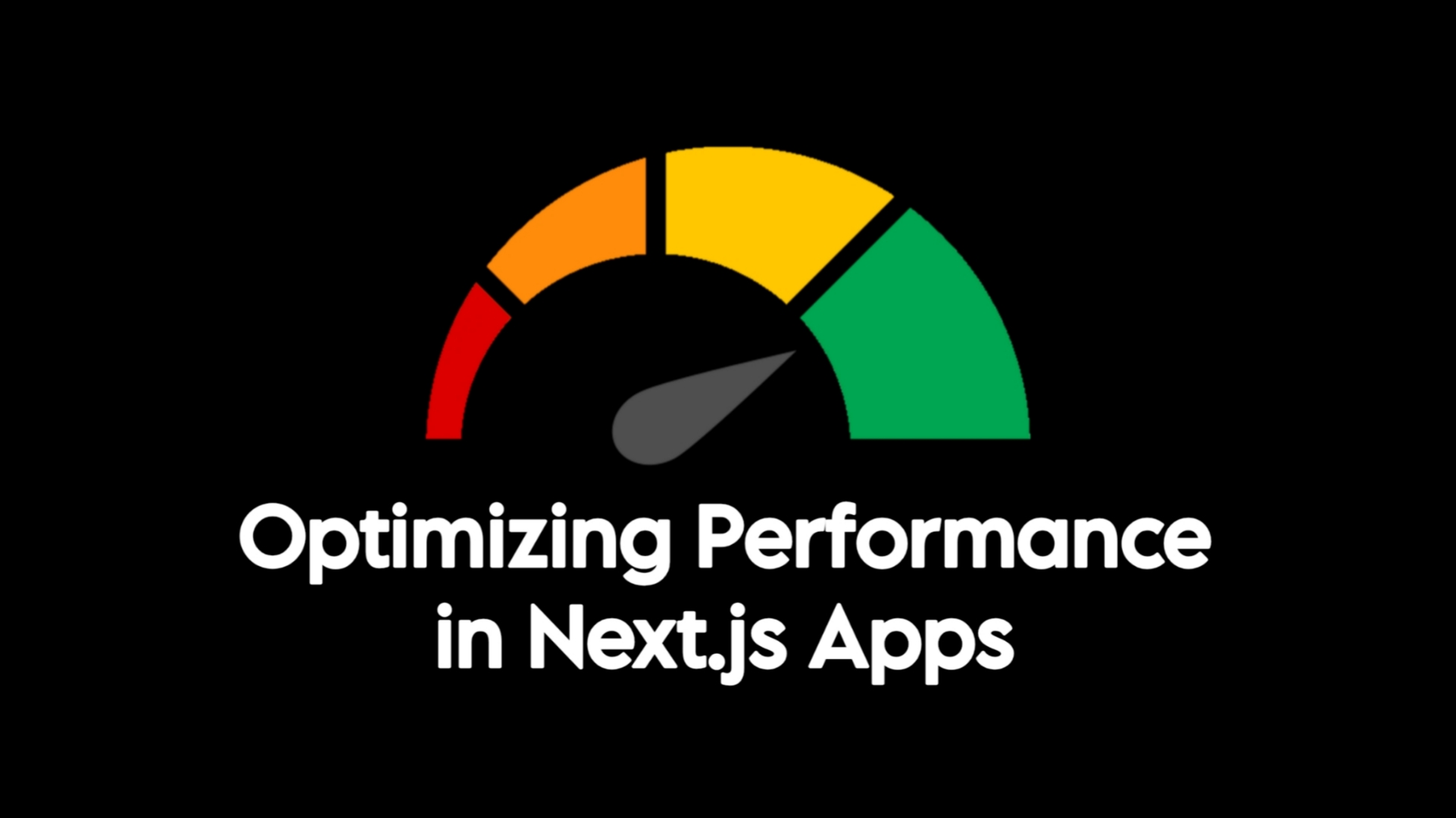
How to Optimize Performance of a Next.js App: Best Practices for Speed and SEO (Next.js 15.2)
In today’s fast-paced digital world, performance is everything. A slow Next.js app can hurt user experience, increase bounce rates, and tank your SEO rankings. Fortunately, Next.js—especially with its latest version, 15.2—offers powerful tools and features to supercharge your app’s speed. In this blog, we’ll dive into proven strategies to optimize your Next.js app for performance, leveraging the latest updates and best practices. Let’s get started!
Why Performance Matters for Next.js Apps
Search engines like Google prioritize fast-loading websites, and users expect near-instant page loads. Poor performance leads to lower rankings, fewer conversions, and frustrated visitors. With Next.js 15.2, released in February 2025, you have cutting-edge features like improved Turbopack performance and streaming metadata to make optimization easier than ever. Here’s how to do it right.
1. Leverage Next.js Image Optimization
Images are often the biggest culprits behind slow load times. The next/image
component in Next.js 15.2 automatically optimizes images by compressing them, converting to modern formats like WebP, and enabling lazy loading.
<Image
src="/example.jpg"
alt="Optimized image for Next.js app"
width={500}
height={300}
placeholder="blur"
/>
Why It Works:
- Reduces file sizes without sacrificing quality.
- Lazy loads images below the fold, improving initial page load.
- Boosts Core Web Vitals (like Largest Contentful Paint), a key SEO factor.
Pro Tip: Always use next/image
over <img>
tags for better performance.
2. Use Server-Side Rendering (SSR) and Static Site Generation (SSG)
Next.js 15.2 shines with its hybrid rendering options: SSR and SSG. These methods pre-render pages, making them instantly available to users and search engines.
When to Use:
- SSG with
getStaticProps
: Ideal for static content like blogs or landing pages.
export async function getStaticProps() {
const data = await fetchData();
return { props: { data } };
}
- SSR with
getServerSideProps
: Perfect for dynamic pages like user profiles.
export async function getServerSideProps() {
const data = await fetchDynamicData();
return { props: { data } };
}
3. Enable Incremental Static Regeneration (ISR)
ISR lets you update static pages without rebuilding your entire app. It’s perfect for content that changes occasionally, like blog posts or product listings.
export async function getStaticProps() {
const data = await fetchData();
return {
props: { data },
revalidate: 60, // Regenerate every 60 seconds
};
}
4. Optimize Third-Party Scripts with next/script
<Script
src="https://example.com/analytics.js"
strategy="lazyOnload"
/>
5. Minimize JavaScript with Code Splitting
const HeavyComponent = dynamic(() => import('../components/HeavyComponent'), {
loading: () => <p>Loading...</p>,
ssr: false,
});
6. Boost Performance with Turbopack (Next.js 15.2)
Next.js 15.2 introduces performance improvements to Turbopack, a Rust-based successor to Webpack. It’s faster and more efficient for builds and hot module replacement (HMR).
next dev --turbo
7. Optimize Fonts for Speed
import { Inter } from 'next/font/google';
const inter = Inter({ subsets: ['latin'] });
8. Monitor and Test Performance
Use tools like Google PageSpeed Insights and Lighthouse to track your app’s performance.
SEO Benefits of a Fast Next.js App
- Faster Load Times: Google rewards sites with low LCP and FCP.
- Better User Experience: Lower bounce rates signal quality content.
- Mobile-Friendly: Next.js’s responsive design aligns with mobile-first indexing.
Conclusion
Optimizing your Next.js app’s performance is a win-win: happier users and better search rankings. Implement these best practices—image optimization, SSR/SSG, ISR, and more—to see real results. Start today, measure your progress, and watch your app soar!