Back to blogs
Setting Up Supabase in a React/Next.js App
March 7, 2025
6 min read
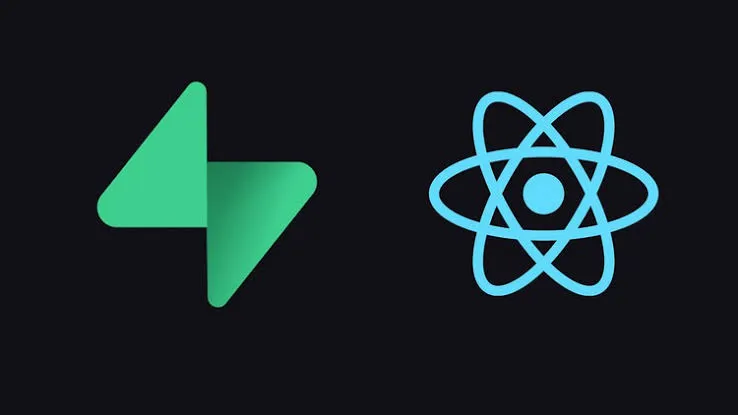
Supabase is an open-source alternative to Firebase, offering a Postgres database, authentication, storage, and real-time capabilities...
Setting Up Supabase in a React/Next.js App
1. Create a Supabase Project:
Sign up at supabase.com and create a new project.
Note the supabaseUrl
and anonKey
from the project settings.
2. Install Supabase SDK:
npm install @supabase/supabase-js
3. Initialize Supabase Client:
import { createClient } from "@supabase/supabase-js";
const supabaseUrl = process.env.NEXT_PUBLIC_SUPABASE_URL;
const supabaseAnonKey = process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY;
export const supabase = createClient(supabaseUrl, supabaseAnonKey);
4. Fetching Data in React:
import { useEffect, useState } from "react";
import { supabase } from "../supabase";
function App() {
const [data, setData] = useState([]);
useEffect(() => {
async function fetchData() {
let { data, error } = await supabase.from("your_table").select("*");
if (!error) setData(data);
}
fetchData();
}, []);
return <div>{JSON.stringify(data)}</div>;
}
export default App;
Supabase SSR in Next.js
Next.js supports server-side rendering (SSR) with Supabase...
import { supabase } from "../supabase";
export async function getServerSideProps() {
let { data, error } = await supabase.from("your_table").select("*");
return {
props: { data: data || [] },
};
}
export default function Page({ data }) {
return <pre>{JSON.stringify(data, null, 2)}</pre>;
}
Benefits of Using Supabase SSR
- ✅ Faster initial page load with pre-fetched data.
- ✅ Improved SEO for content-heavy pages.
- ✅ Avoids client-side fetch delays for better user experience.
Pros and Cons of Supabase
✅ Pros
- PostgreSQL-based: A full relational database with SQL support.
- Real-time capabilities: Built-in real-time database subscriptions.
- Authentication: Easy-to-use auth system with providers like Google, GitHub, etc.
- Serverless & Scalable: Works well with serverless architectures like Vercel.
- Free Tier Available: Generous free plan for small projects.
❌ Cons
- Cold start delays: Free-tier projects may experience latency after periods of inactivity.
- Limited Edge Functions: Compared to Firebase’s Cloud Functions.
- Self-hosting complexity: Running Supabase on your own infrastructure requires extra setup.
- Pricing concerns: Can become costly for high-traffic applications.
Conclusion
Supabase is a fantastic backend for React and Next.js apps...
SupabaseReactNext.jsBackend